Knowing how to get column names in a PostgreSQL database is a fundamental skill for any data professional. Being able to quickly and accurately identify the structure of your database can help you write more efficient SQL queries, validate the integrity of your data, and document your schema for better collaboration and maintenance.
In this blog post, we’ll walk through the step-by-step process of retrieving column names in PostgreSQL, starting with the basics of database structure and then exploring the various SQL techniques you can use.
PostgreSQL 101: The Basics of Database Tables and Columns
At the core of any PostgreSQL database are tables, which are the fundamental units for storing and organizing data.
Each table consists of one or more columns, which represent the different types of information you want to store. For example, a “users” table might have columns for a user’s name, email address, and registration date.
Understanding the structure of your database tables and the names of their columns is crucial for effective data management and querying. Without this knowledge, you may struggle to write accurate SQL statements, leading to errors, inefficient queries, and potentially incorrect results.
Some key points to keep in mind about PostgreSQL tables and columns:
- Tables: These are the containers that hold your data, similar to spreadsheets or Excel worksheets.
- Columns: These are the individual fields within a table, representing the different types of information you want to store, such as names, dates, or numerical values.
- Data Types: Each column has a specific data type, such as text, integer, or timestamp, which determines the kind of data it can hold.
- Schema: The overall structure of your database, including the names and relationships of your tables and columns, is known as the database schema.
Get Column Names in PostgreSQL with SQL
Now that you have a solid understanding of PostgreSQL tables and columns, let’s dive into the various SQL techniques you can use to list the column names in your database.
Using the information_schema.columns View
One of the most straightforward ways to retrieve column names in PostgreSQL is by querying the information_schema.columns view. This view provides a comprehensive list of all the columns in your database, along with their associated table names, data types, and other metadata.
Here’s an example SQL query to list all the column names for a specific table:
SELECT column_name, data_type
FROM information_schema.columns
WHERE table_name = ‘your_table_name’;
This query will return a result set with two columns: column_name and data_type. The column_name column will contain the names of all the columns in the specified table, and the data_type column will show the data type of each column.
You can also use the information_schema.columns view to retrieve column names across multiple tables by adding a WHERE clause to filter the results:
SELECT table_name, column_name, data_type
FROM information_schema.columns
WHERE table_schema = ‘public’
AND table_name IN (‘table1’, ‘table2’, ‘table3’);
This query will return the column names and data types for the specified tables within the public schema.
Using the d Command in the PostgreSQL CLI
If you prefer to work directly in the PostgreSQL command-line interface (CLI), you can use the d command to list the columns in a specific table. This command is part of the psql utility, which is the default PostgreSQL interactive terminal.
To list the columns for a table, simply type d table_name in the psql prompt. This will display the table’s structure, including the column names and their data types.
For example:
d users
This will show the column names and data types for the users table.
You can also use the d+ command to get more detailed information about the table, including any constraints or indexes that have been defined.
Get Column Names in PostgreSQL: The Programming Approach
While the SQL-based approach we covered in the previous section is straightforward for retrieving column names, there are times when a programmatic approach may be more suitable.
By integrating column name retrieval into your application code, you can dynamically generate queries, streamline data processing, and enhance the overall functionality of your PostgreSQL-powered systems.
Here’s an example of how you can use Psycopg2 to fetch column names from a PostgreSQL table:
import psycopg2
# Connect to the PostgreSQL database
conn = psycopg2.connect(
host=”your_host”,
database=”your_database”,
user=”your_username”,
password=”your_password”
)
# Create a cursor object
cur = conn.cursor()
# Retrieve column names for a specific table
table_name = “your_table_name”
cur.execute(f”SELECT * FROM {table_name} LIMIT 0″)
column_names = [desc[0] for desc in cur.description]
print(column_names)
# Close the connection
conn.close()
In this example, we first establish a connection to the PostgreSQL database using the psycopg2.connect() function. We then create a cursor object, which allows us to execute SQL queries and retrieve the results.
To fetch the column names, we use the cur.execute() method to execute a SELECT * FROM query on the target table, but with a LIMIT 0 clause to prevent the actual retrieval of any data. The cur.description attribute contains information about the columns in the result set, including the column names. We then use a list comprehension to extract the column names from the cur.description list.
Finally, we close the database connection.
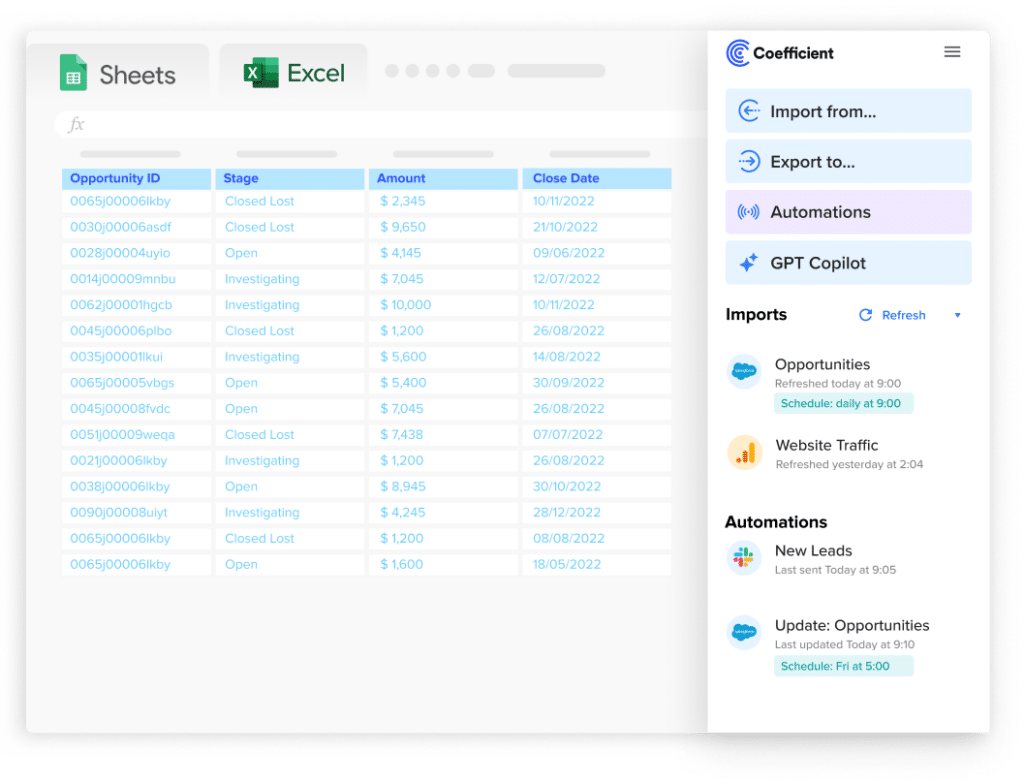
Stop exporting data manually. Sync data from your business systems into Google Sheets or Excel with Coefficient and set it on a refresh schedule.
Get Started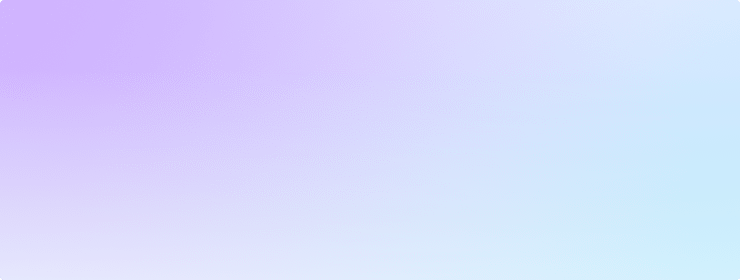
The programmatic approach offers several benefits over the SQL-based method:
- Dynamic Query Generation: By retrieving column names programmatically, you can dynamically generate SQL queries based on the available columns in your tables. This can be particularly useful when building flexible, scalable applications that need to adapt to changes in the database schema.
- Seamless Integration with Application Code: Integrating column name retrieval into your application code allows you to easily incorporate this functionality into your data processing workflows, reporting tools, or other application-level features.
- Improved Maintainability: When column names change in your database, you can update the relevant parts of your application code without having to manually modify SQL queries or other hard-coded references to column names.
Get Column PostgresSQL Column Names from Your Spreadsheet
While the SQL-based and programmatic approaches we’ve covered so far can be effective, they still require a certain level of manual effort and technical expertise. If you’re looking for a more streamlined way to retrieve column names from your PostgreSQL database, consider using Coefficient.
Coefficient allows you to query your database directly from Excel or Google Sheets, offering three options:
- Tables & Columns Import: Select specific tables and columns to import.
- Custom SQL Query Import: Write SQL queries for complex data pulls.
- GPT SQL Builder: Generate SQL queries using plain English.
When setting up a new import in Coefficient, the platform automatically fetches schema information, including column names, for your tables. This feature allows you to quickly visualize your database structure and select the necessary columns for data processing tasks.
Let’s explore the Custom SQL Query Import method, which offers maximum flexibility for data teams:
Open Coefficient’s sidebar and select “Import from.”
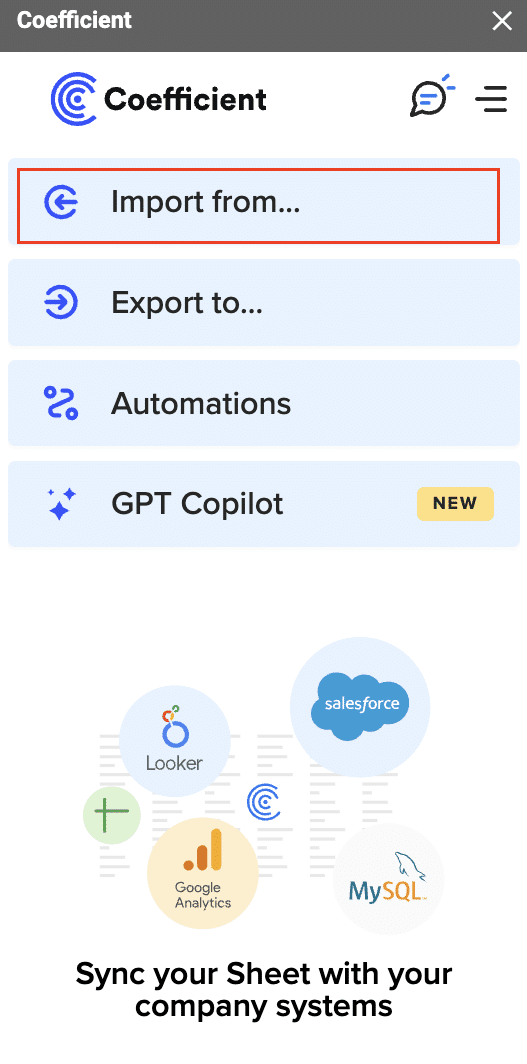
Select “PostgreSQL” from the list.

Choose “From Tables & Columns”.
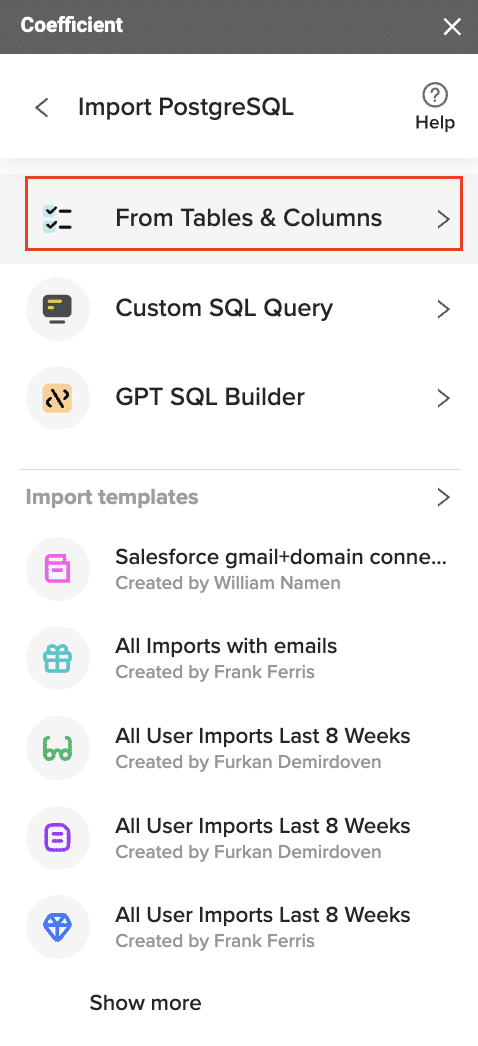
The Import Preview window opens showing all the table schemas from your PostgreSQL database. Select the table for your import. (eg. ”coeff.actor”)
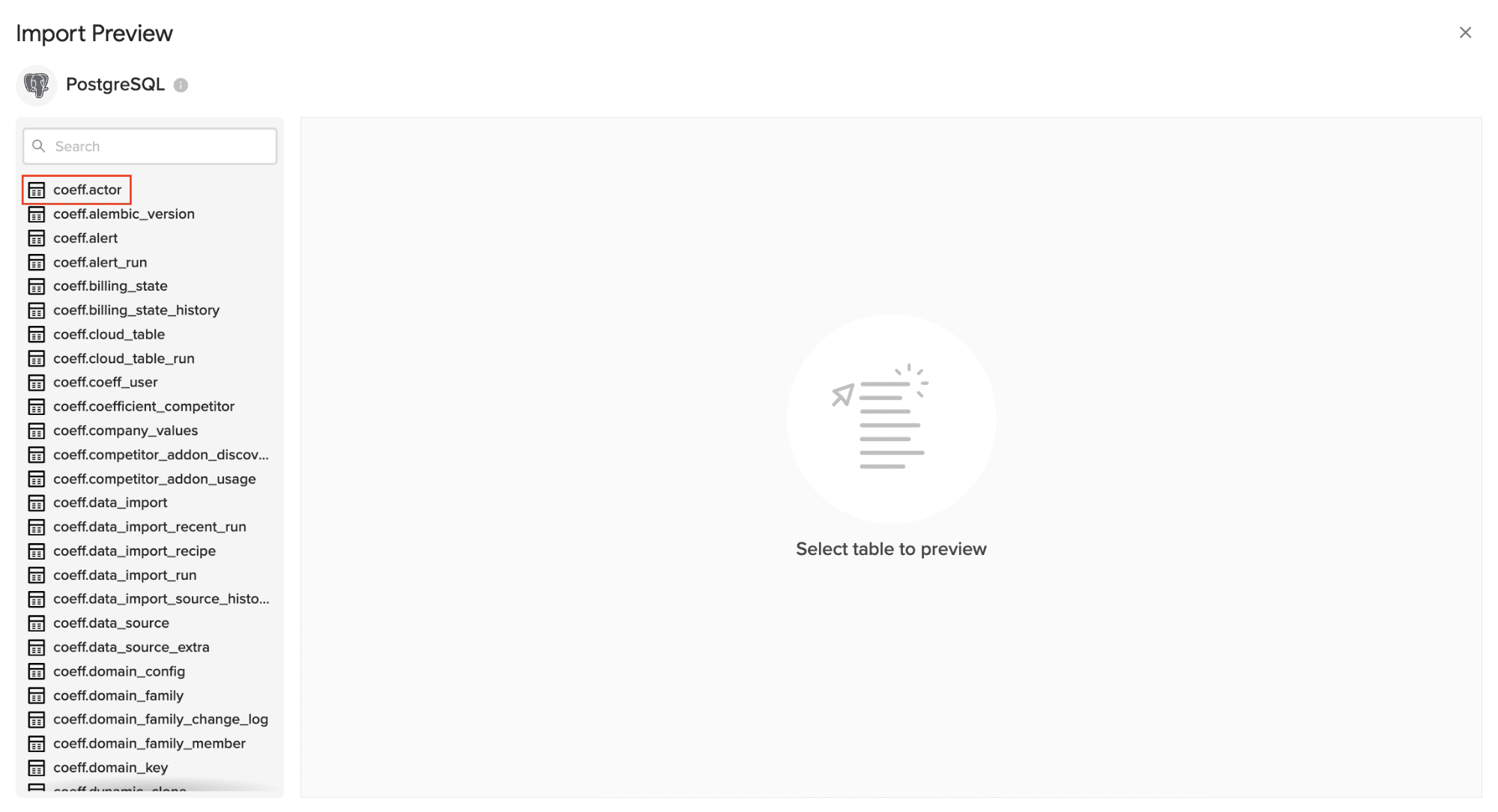
Once the table is selected, the fields within that table will appear in a list on the left side of the Import Preview window. Select the fields you want to include in your import by checking/unchecking the corresponding boxes.
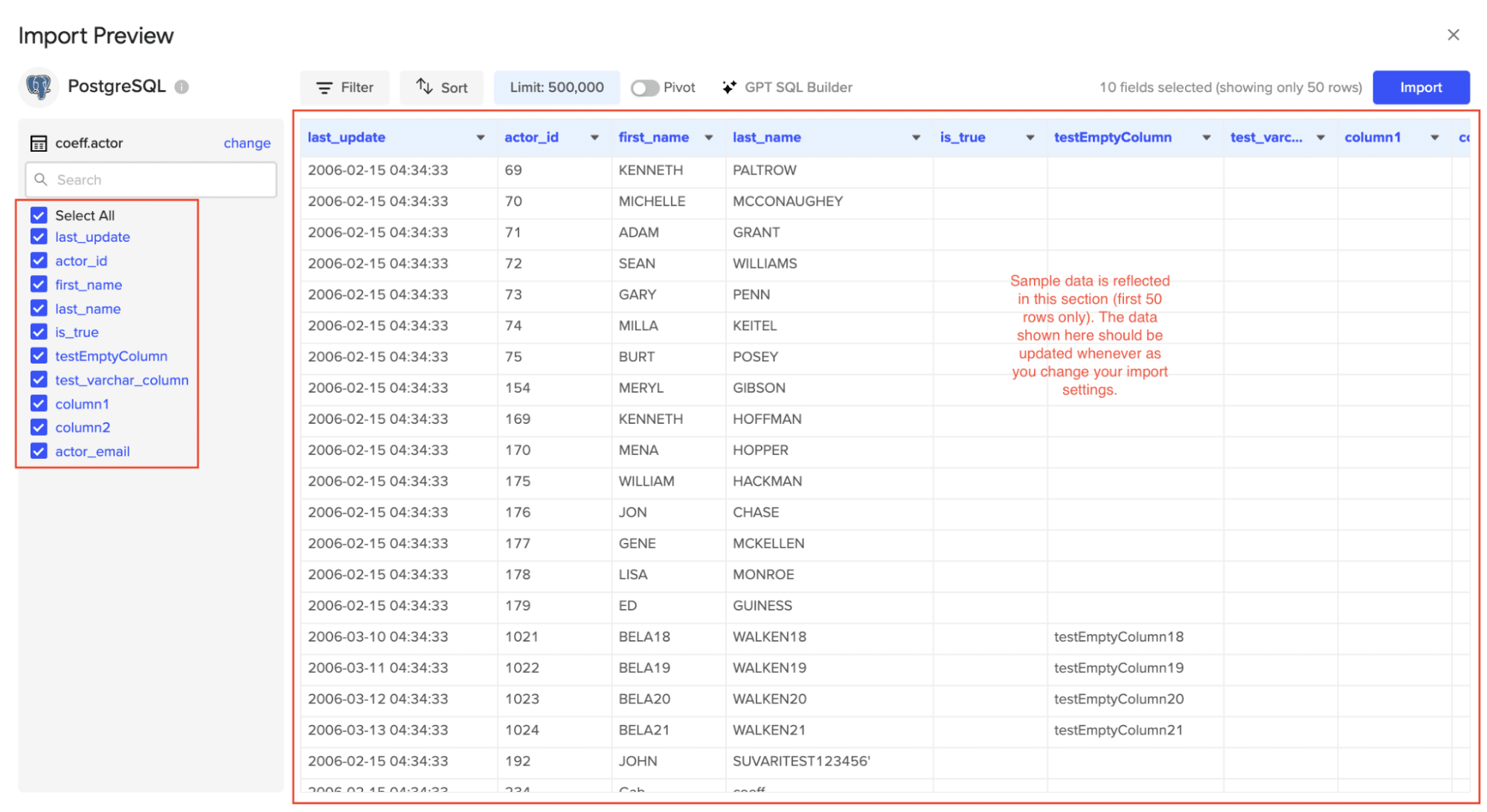
Customize your import by adding filters, sorts, limits, or even grouping the data into a cloud pivot table. Then “Import” when done.
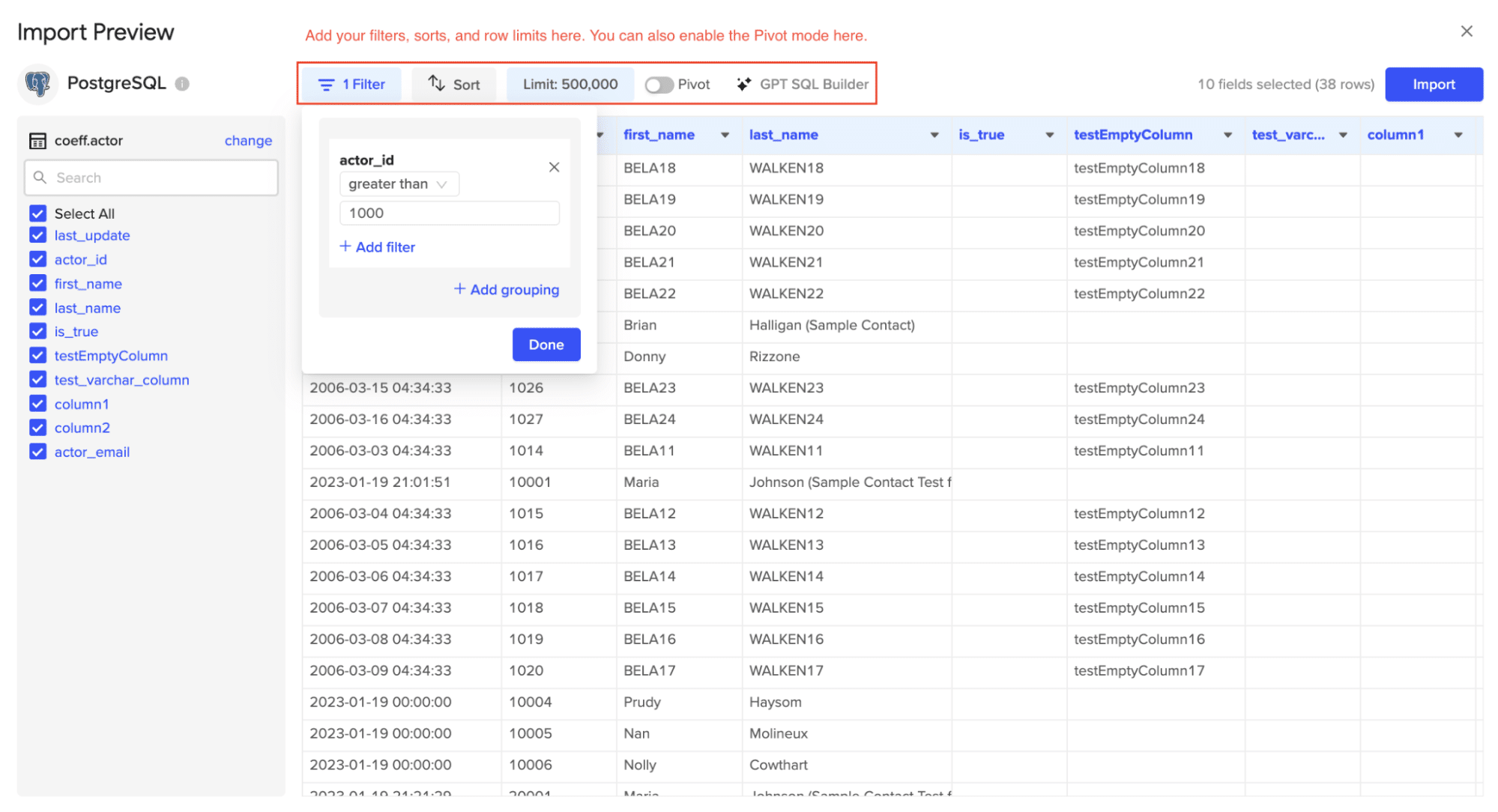
Your PostgreSQL import will automatically populate your spreadsheet in a few seconds!
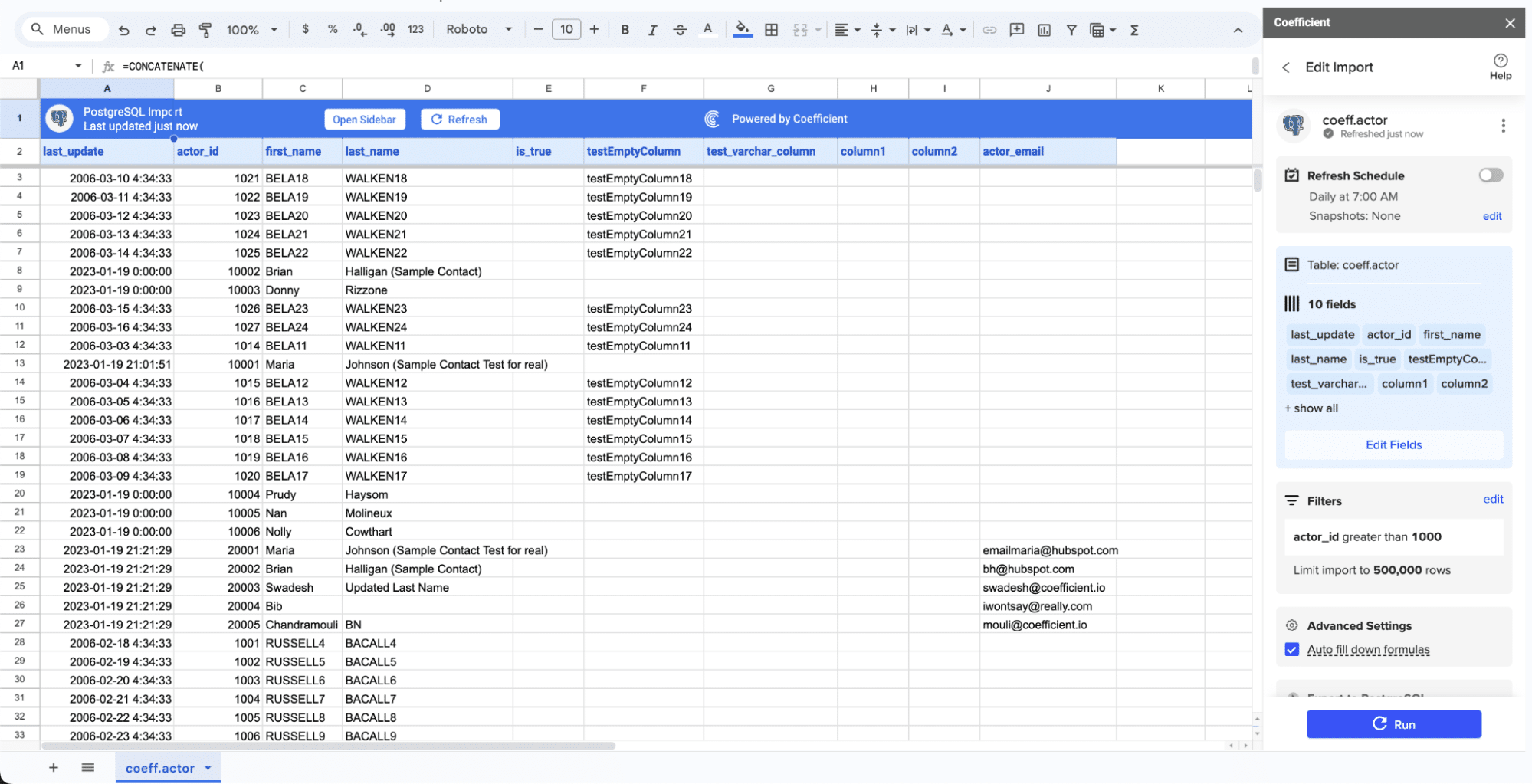
Advanced Techniques: Exploring Column Names Across Multiple Tables
In some cases, you may need to go beyond retrieving column names for a single table and instead explore column names across an entire PostgreSQL database. This can be useful for tasks such as schema documentation, data lineage analysis, or identifying potential data integration issues.
To find columns with a specific name across all tables in a PostgreSQL database, you can query the information_schema.columns view. This view provides detailed information about the columns in your database, including the table name, column name, data type, and more.
Here’s an example SQL query that retrieves all column names matching a specific pattern (e.g., “created_at”) across your database:
SELECT
table_name,
column_name
FROM
information_schema.columns
WHERE
column_name LIKE ‘%created_at%’
AND table_schema = ‘public’
ORDER BY
table_name, column_name;
This query searches the information_schema.columns view for any column names that contain the phrase “created_at”, and returns the table name and column name for each match. The table_schema = ‘public’ condition ensures that we only consider tables in the public schema (you can modify this to include other schemas if needed).
By using this advanced technique, you can quickly identify all the tables and columns in your PostgreSQL database that match a specific naming pattern. This can be particularly useful for:
- Schema Documentation: Generating comprehensive documentation of your database schema, including all available columns and their locations.
- Data Lineage Analysis: Tracing the flow of data through your system by identifying where specific columns are used across multiple tables.
- Data Validation: Ensuring that critical columns (e.g., unique identifiers, timestamps) are consistently named and available across your database.
Mastering PostgreSQL Data
In this comprehensive guide, we’ve explored the various methods for retrieving column names in PostgreSQL, from simple SQL queries to more advanced programmatic approaches. We’ve also discussed how to leverage the information_schema.columns view to explore column names across multiple tables, and how tools like Coefficient can streamline this process.
By applying the techniques and strategies covered in this post, you’ll be able to enhance the efficiency, productivity, and data governance of your PostgreSQL-based applications. Whether you’re a data engineer, database administrator, or application developer, mastering column name retrieval will be a valuable skill that can help you unlock the full potential of your data.
Remember, Coefficient (https://coefficient.io/get-started) is a powerful tool that can simplify many of the data management tasks we’ve discussed, including connecting to your PostgreSQL databases, visualizing schema information, and selecting the necessary columns for your data processing workflows. Consider giving Coefficient a try to see how it can streamline your data management efforts and help you focus on delivering more value to your organization.