Google Sheets API Tutorial: How to Read & Write Data [Python, JavaScript, or No Code]
In this tutorial, we’ll explore the fundamentals of the Google Sheets API, including how to set it up and perform basic read and write operations.
We’ll provide code samples in both Python and JavaScript, so you can choose the language that best fits your needs.
By the end of this post, you’ll have a solid understanding of how to leverage the Google Sheets API to streamline your data management processes.
Google Sheets API Fundamentals
The Google Sheets API is a RESTful API that allows you to interact with Google Sheets programmatically. It provides a set of methods and endpoints that enable you to perform various operations, such as:
- Reading and writing cell data
- Updating and formatting spreadsheets
- Inserting, deleting, and moving sheets
- Applying filters and sorting data
- Retrieving metadata about a spreadsheet
To use the Google Sheets API, you’ll need to authenticate your application and obtain the necessary credentials. This is typically done by creating a project in the Google Cloud Console and enabling the Google Sheets API for that project.
The API supports a range of authentication methods, including OAuth 2.0, which allows users to grant your application access to their Google Sheets data without sharing their login credentials. This makes the API suitable for both personal and enterprise-level use cases.
It’s important to note that the Google Sheets API has certain limits and quotas, such as the number of requests per day or the size of the data you can retrieve. Understanding these limits and planning your usage accordingly is crucial to ensure a smooth and reliable integration.
How to Use Google Sheets API 101: Understanding the Basics
To start using the Google Sheets API, you’ll need to follow these steps:
- Enable the Google Sheets API: Log in to the Google Cloud Console and create a new project. Then, navigate to the “APIs & Services” section and enable the Google Sheets API for your project.
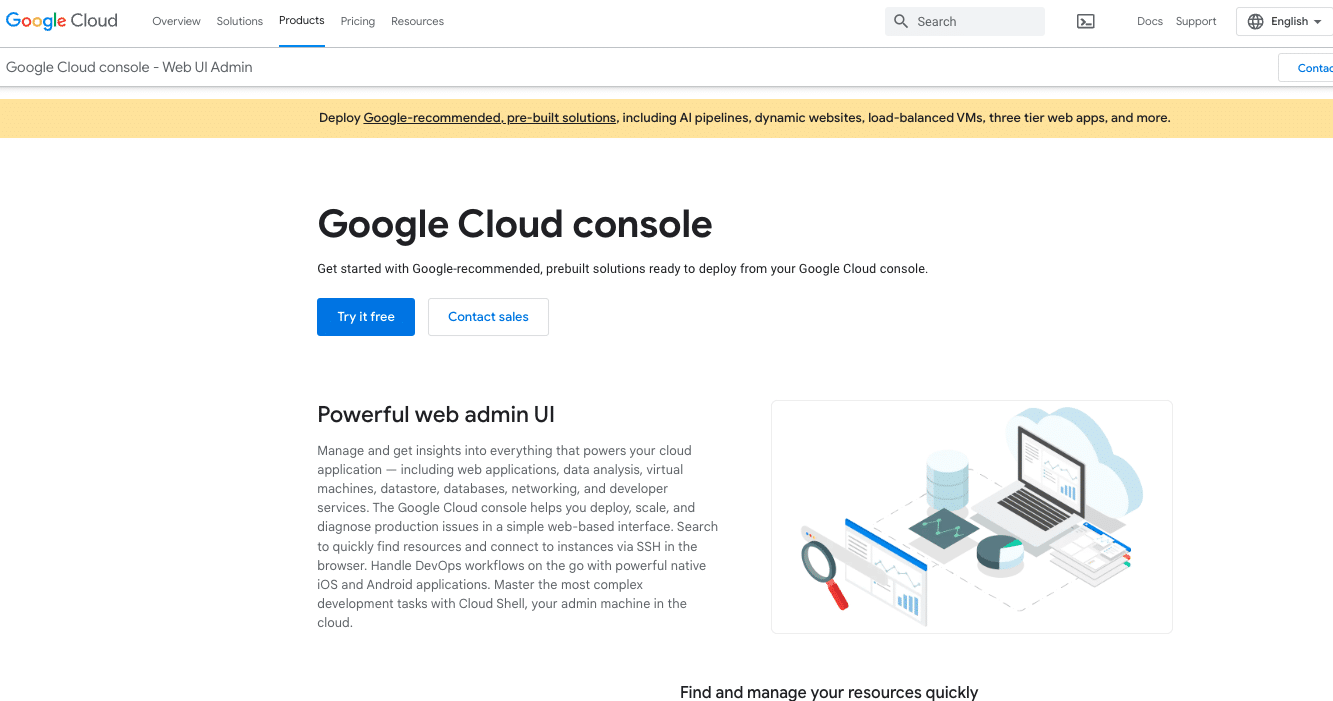
- Create Credentials: In the “APIs & Services” section, go to the “Credentials” tab and create the necessary credentials for your application, such as an API key or OAuth client ID and client secret.
- Set up Authentication: Depending on the authentication method you choose, you’ll need to configure your application to handle the authentication process. This may involve implementing OAuth 2.0 flows or using the API key for simple API access.
Once you have the necessary credentials and authentication set up, you can start writing code to interact with the Google Sheets API. Here’s a quick start example in Python:
Python Example
from googleapiclient.discovery import build
from google.oauth2 import service_account
# Set up credentials
creds = service_account.Credentials.from_service_account_info(
info={
“type”: “service_account”,
“project_id”: “your-project-id”,
“private_key_id”: “your-private-key-id”,
“private_key”: “your-private-key”,
“client_email”: “your-client-email”,
“client_id”: “your-client-id”,
“auth_uri”: “https://accounts.google.com/o/oauth2/auth”,
“token_uri”: “https://oauth2.googleapis.com/token”,
“auth_provider_x509_cert_url”: “https://www.googleapis.com/oauth2/v1/certs”,
“universe_domain”: “googleapis.com”
}
)
# Create the Sheets API client
sheets_service = build(‘sheets’, ‘v4’, credentials=creds)
# Read data from a spreadsheet
spreadsheet_id = ‘your-spreadsheet-id’
range_name = ‘Sheet1!A1:B10’
result = sheets_service.spreadsheets().values().get(
spreadsheetId=spreadsheet_id, range=range_name).execute()
values = result.get(‘values’, [])
# Write data to a spreadsheet
new_values = [[‘Name’, ‘Age’], [‘John’, ’30’], [‘Jane’, ’25’]]
body = {‘values’: new_values}
result = sheets_service.spreadsheets().values().update(
spreadsheetId=spreadsheet_id, range=range_name,
valueInputOption=’USER_ENTERED’, body=body).execute()
And here’s the same example in JavaScript:
JavaScript Example
const { google } = require(‘googleapis’);
// Set up credentials
const auth = new google.auth.GoogleAuth({
credentials: {
client_email: ‘your-client-email’,
private_key: ‘your-private-key’,
},
scopes: [‘https://www.googleapis.com/auth/spreadsheets’],
});
// Create the Sheets API client
const sheets = google.sheets({ version: ‘v4’, auth });
// Read data from a spreadsheet
const spreadsheetId = ‘your-spreadsheet-id’;
const range = ‘Sheet1!A1:B10’;
const response = await sheets.spreadsheets.values.get({
spreadsheetId,
range,
});
const values = response.data.values || [];
// Write data to a spreadsheet
const newValues = [[‘Name’, ‘Age’], [‘John’, ’30’], [‘Jane’, ’25’]];
const writeResponse = await sheets.spreadsheets.values.update({
spreadsheetId,
range,
valueInputOption: ‘USER_ENTERED’,
requestBody: { values: newValues },
});
In both examples, we first set up the necessary credentials to authenticate with the Google Sheets API. Then, we create the API client and use it to read data from a specific range in a spreadsheet, as well as write new data to the same range.
These examples should give you a good starting point for working with the Google Sheets API. In the next part of this tutorial, we’ll dive deeper into more advanced read and write operations, including handling errors, managing permissions, and exploring additional API features.
Creating and Updating Spreadsheets
Once you’ve mastered the basics of reading data from Google Sheets, the next step is to learn how to create and update spreadsheets programmatically. This opens up a world of possibilities, allowing you to automate tasks, generate reports, and integrate your data with other applications.
To create a new spreadsheet, you’ll use the spreadsheets.create() method. This method takes in a spreadsheet object with properties like the title, properties, and sheets.
Here’s an example in Python:
from googleapiclient.discovery import build
# Create a new spreadsheet
spreadsheet = {
‘properties’: {
‘title’: ‘My New Spreadsheet’
}
}
service = build(‘sheets’, ‘v4’)
result = service.spreadsheets().create(body=spreadsheet).execute()
print(f’Spreadsheet ID: {result.get(“spreadsheetId”)}’)
To update an existing spreadsheet, you’ll use the spreadsheets.update() method. This allows you to modify the spreadsheet’s properties, add or remove sheets, and more. Here’s an example of renaming a sheet in JavaScript:
const { google } = require(‘googleapis’);
const sheets = google.sheets({ version: ‘v4’ });
async function renameSheet(spreadsheetId, sheetId, newSheetName) {
const request = {
spreadsheetId,
resource: {
requests: [
{
updateSheetProperties: {
properties: {
sheetId,
title: newSheetName
},
fields: ‘title’
}
}
]
}
};
const response = await sheets.spreadsheets.batchUpdate(request);
console.log(`Sheet ‘${newSheetName}’ updated successfully.`);
}
These examples should give you a good starting point for creating and updating spreadsheets using the Google Sheets API. Remember to handle errors and edge cases appropriately, and consider adding features like automatic sheet naming or formatting to make your applications more robust.
How to Use Google Sheets API Without a Line of Code
The Google Sheets API is a powerful tool, but it requires coding skills to use effectively. For those who don’t code or prefer a simpler approach, Coefficient’s Any API feature offers an easy-to-use alternative. This tool works alongside the Google Sheets API, allowing anyone to connect their spreadsheets with external data sources, regardless of their technical background.
Three Key Benefits of Coefficient’s Any API
- Simplified Data Import: Pull data from various APIs directly into your sheets without writing complex code. This makes it easy to aggregate data from multiple sources in one place.
- Automatic Refreshes: Set up scheduled refreshes to keep your data up-to-date without the need for cron jobs or scripts. This ensures your sheets always have the latest information.
- User-Friendly Interface: Configure API connections, handle authentication, and manage data mapping through an intuitive point-and-click interface. This democratizes data integration, making it accessible to both citizen analysts and seasoned developers.
How to Use Coefficient’s Any API Feature
In your Google Sheet, open the Coefficient sidebar and click “Import from…”
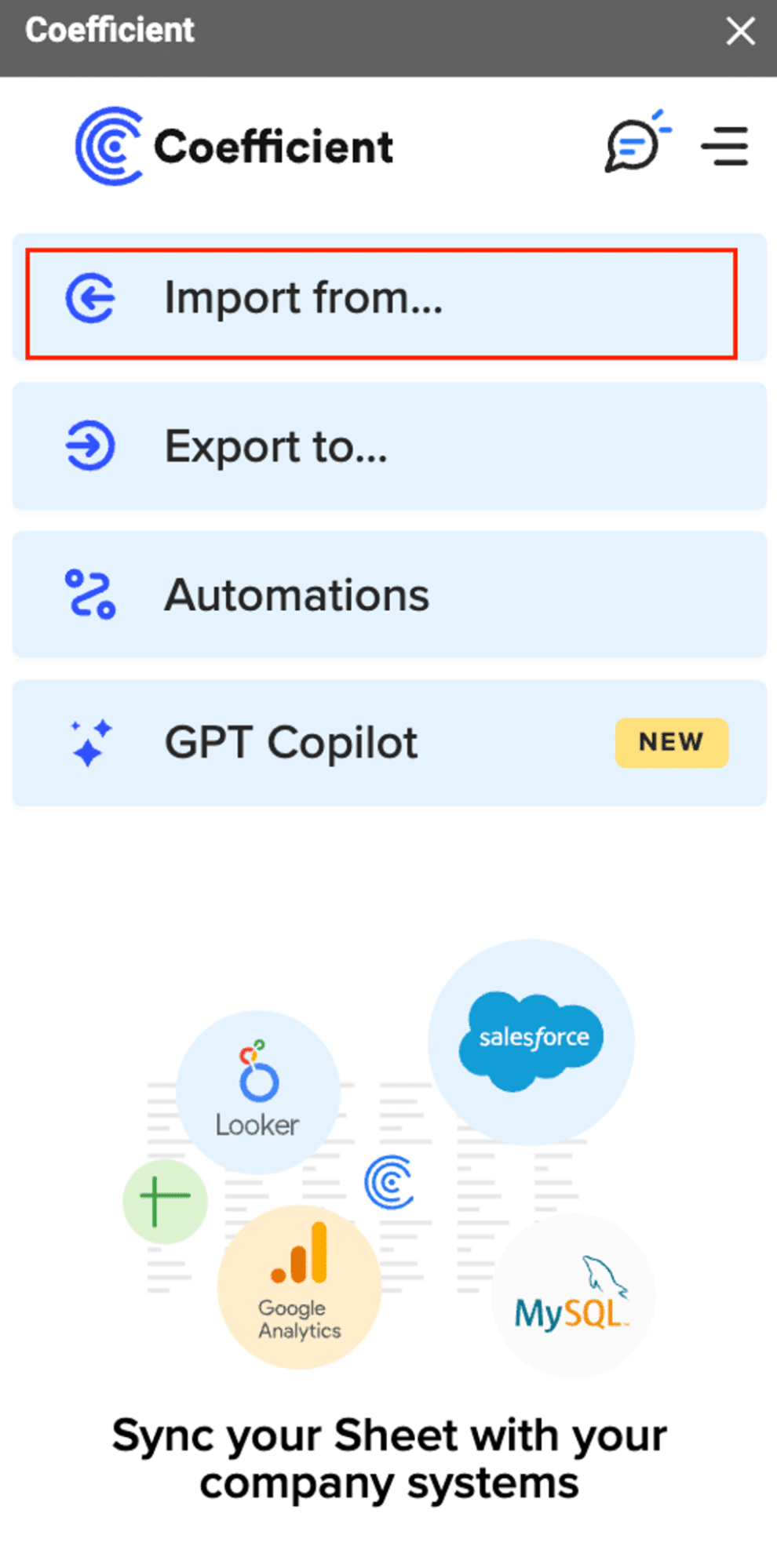
Select “Connect Any API.”
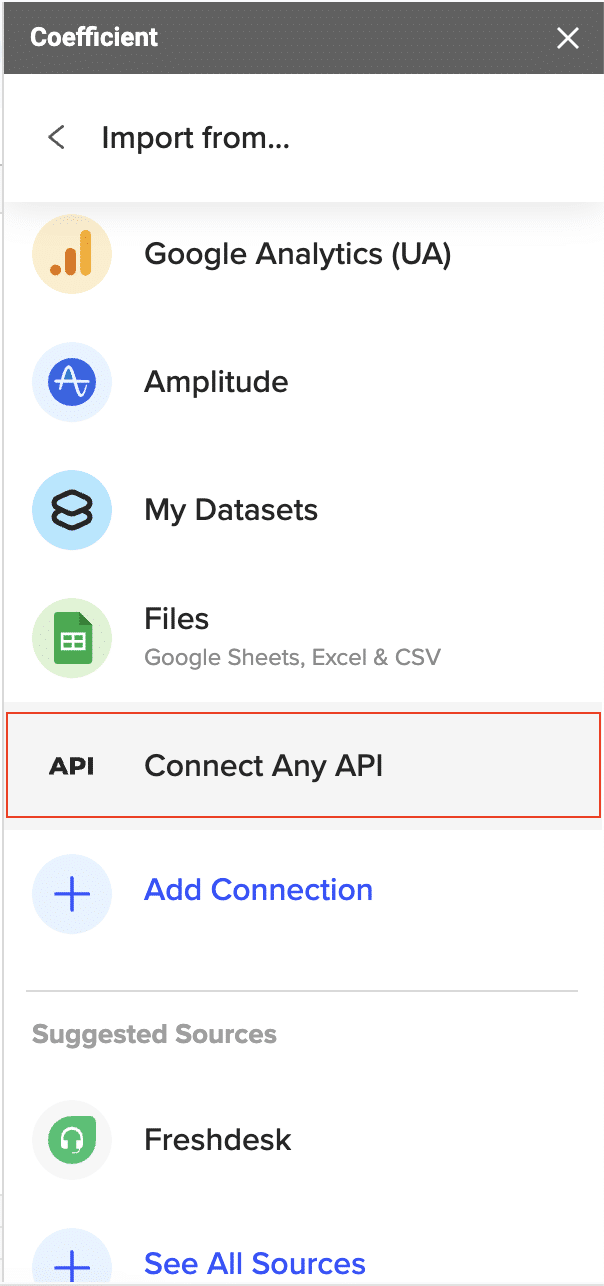
Click “Start from Scratch”
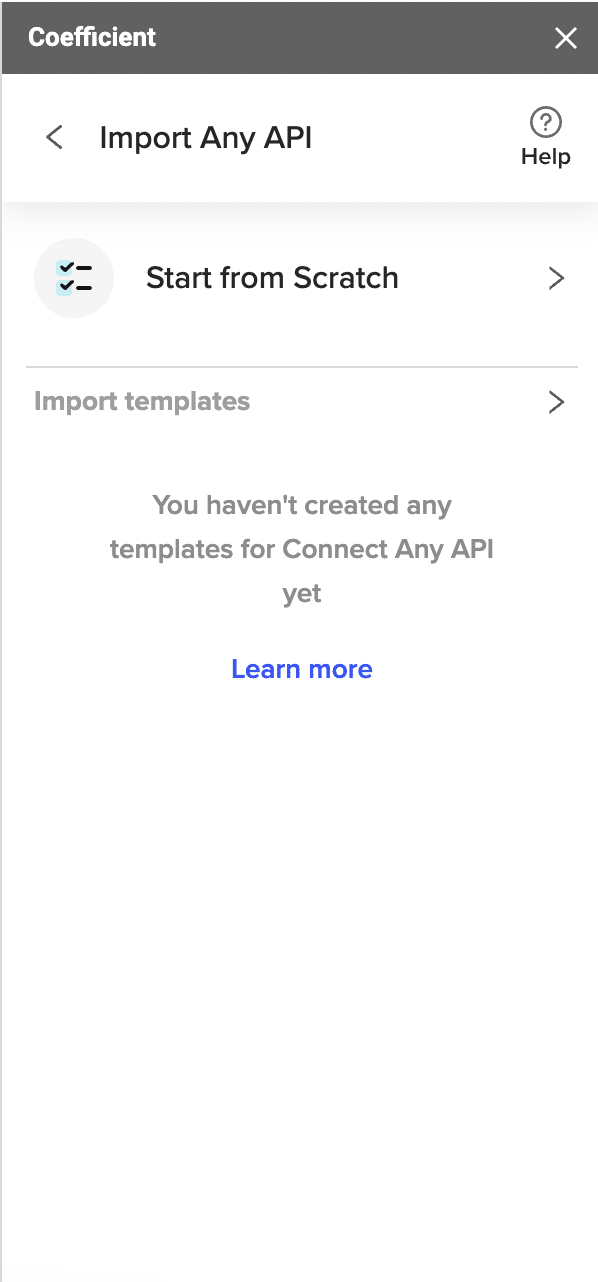
Enter the information needed for your API call.
- Endpoint URL: ttps://pro-api.coinmarketcap.com/v1/cryptocurrency/listings/latest
- Header Key: X-CMC_PRO_API_KEY
- Header Value: API copied from our account.
- Query Parameter Key: cryptocurrency_type
- Query Parameter Value: coins
Click “Preview” to see the data.
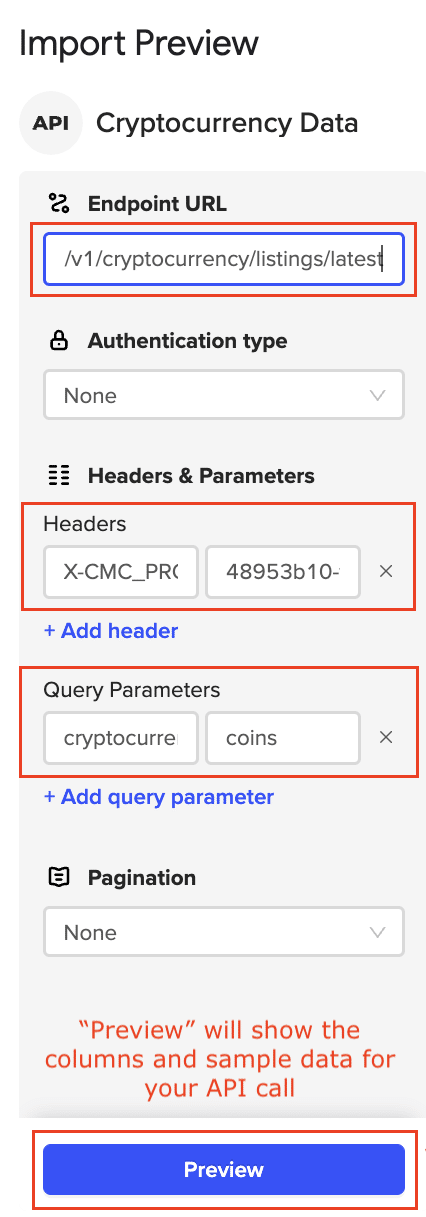
When you click “Preview,” your API call will return the fields/columns. You can select or deselect the necessary fields for your import.
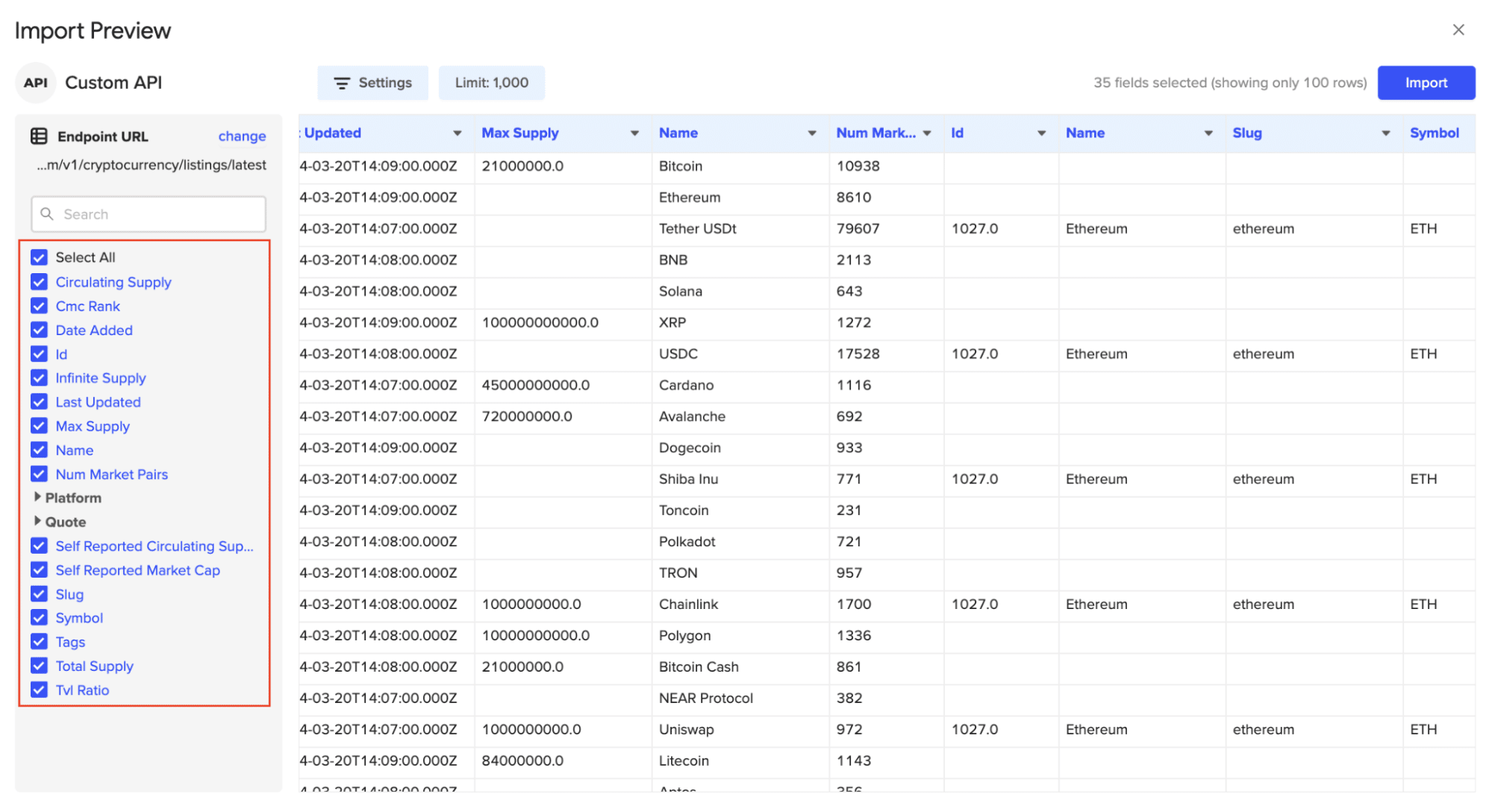
Once you’ve set up your Connect Any API import settings and checked the sample data, click the Import button (located at the top right corner).
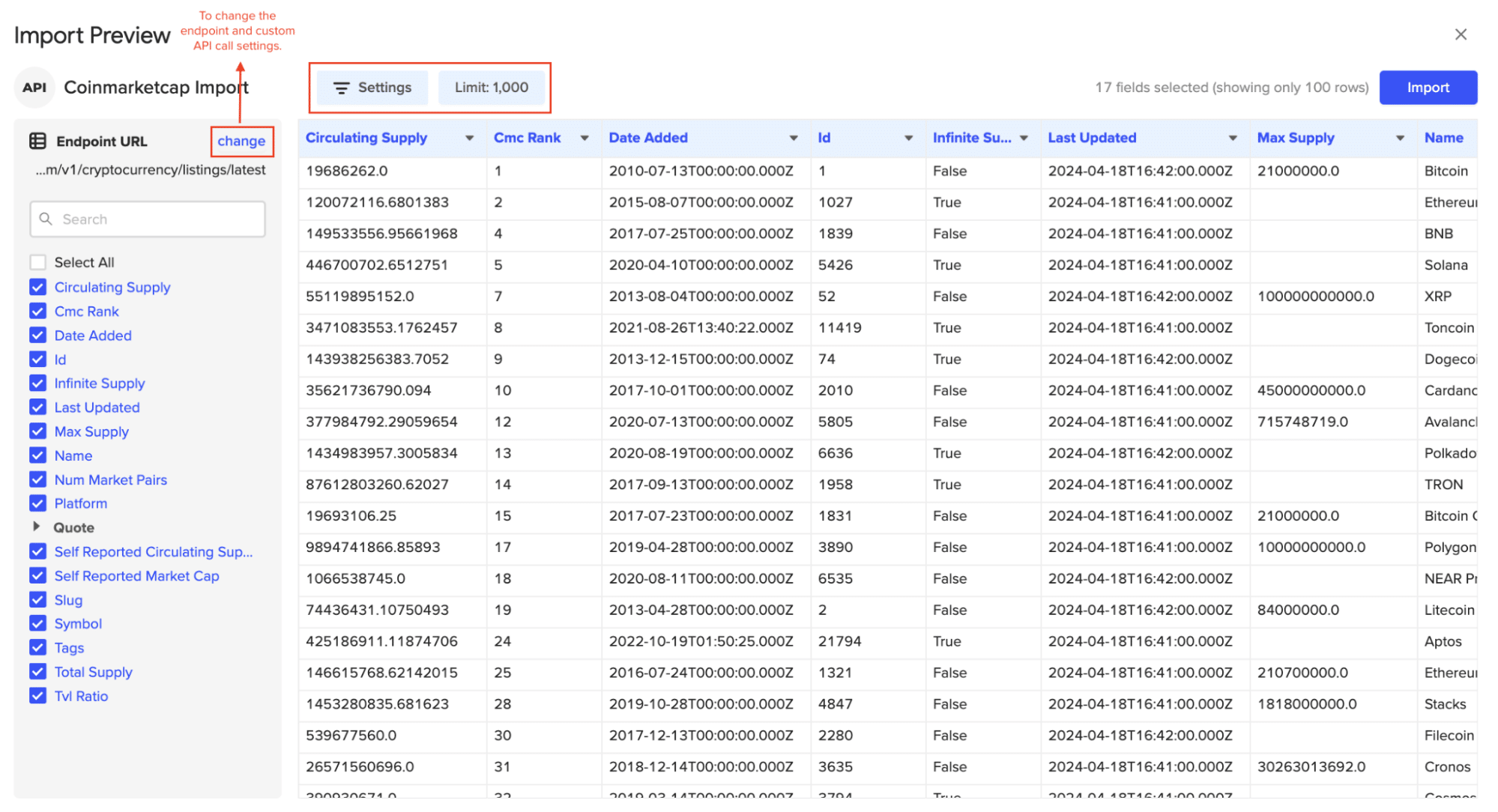
Now you have live cryptocurrency data in your Google Sheet, ready for analysis or integration with other data sources!
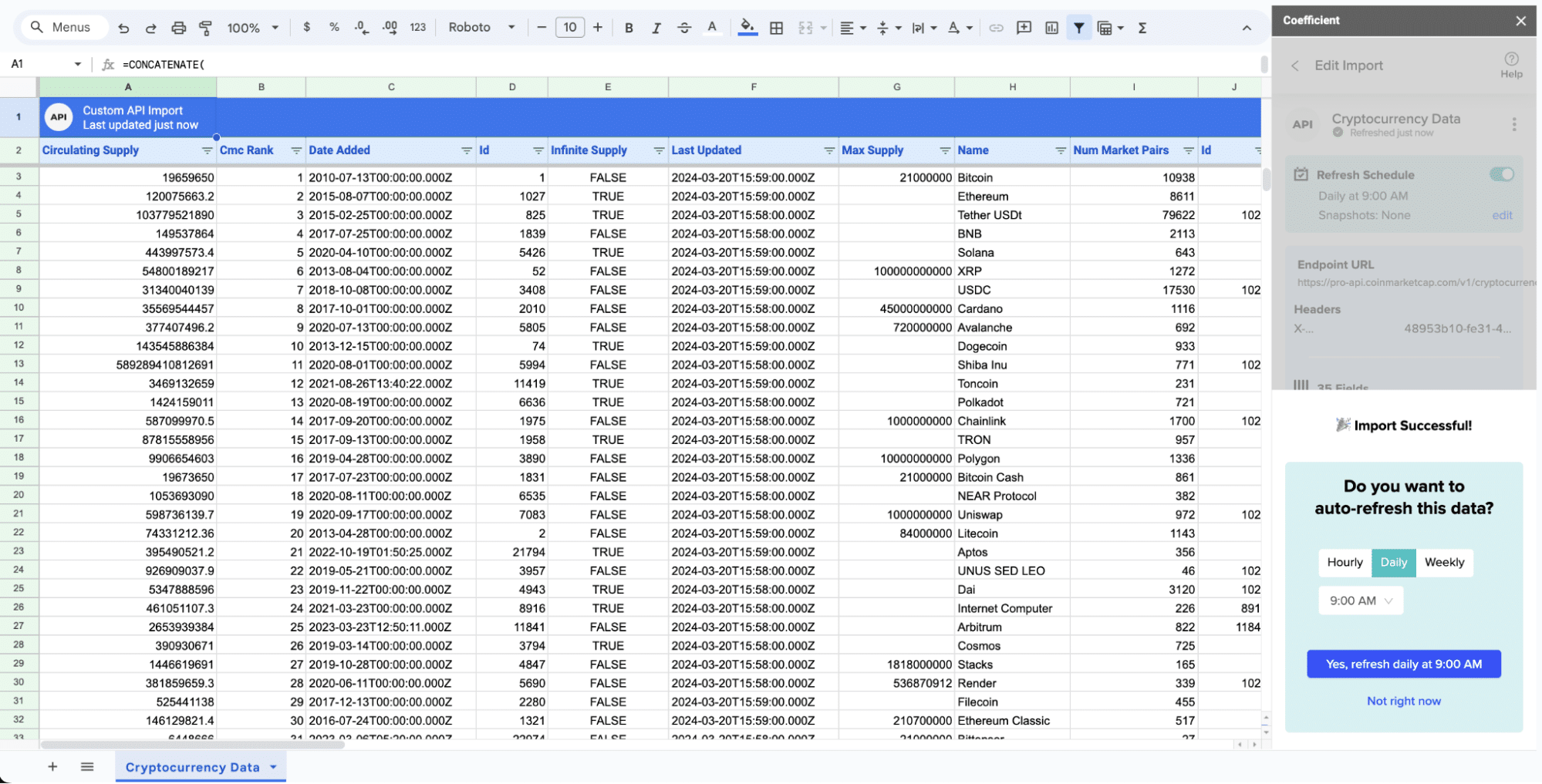
Integrating the Google Sheets API with Other Tools
One of the most powerful aspects of the Google Sheets API is its ability to integrate with other tools and services. By combining the API with other APIs and platforms, you can create powerful data-driven applications that automate workflows, generate reports, and more.
Here are a few examples of integrations you can build:
- CRM Integration: Sync customer data between your CRM and Google Sheets, allowing you to generate custom reports and dashboards.
- Accounting Integration: Automatically import financial data from your accounting software into Google Sheets for analysis and reporting.
- Project Management Integration: Integrate your project management tool with Google Sheets to track progress, generate Gantt charts, and share updates with stakeholders.
- Ecommerce Integration: Pull sales data from your ecommerce platform into Google Sheets for inventory management, forecasting, and performance analysis.
Here’s an example of how you might integrate the Google Sheets API with the Trello API to create a project management dashboard:
const { google } = require(‘googleapis’);
const { Trello } = require(‘@trello/client’);
async function createProjectDashboard(spreadsheetId, trelloApiKey, trelloApiToken) {
const sheets = google.sheets({ version: ‘v4’ });
const trello = new Trello(trelloApiKey, trelloApiToken);
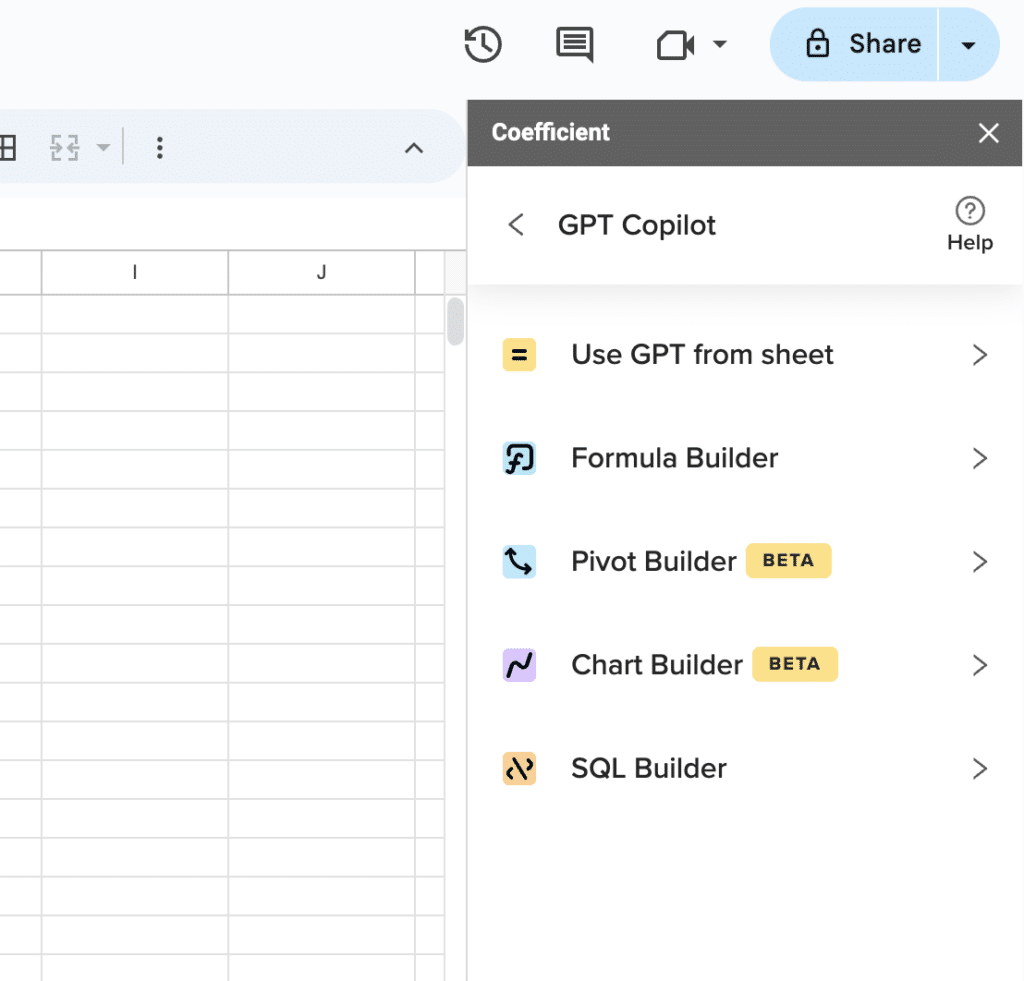
Supercharge your spreadsheets with GPT-powered AI tools for building formulas, charts, pivots, SQL and more. Simple prompts for automatic generation.
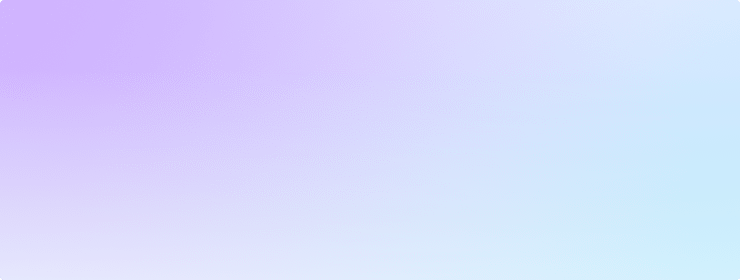
// Fetch data from Trello
const boards = await trello.getBoards();
const cards = await trello.getCards();
// Write data to Google Sheets
const values = [
[‘Board Name’, ‘Card Name’, ‘Due Date’, ‘Status’],
…boards.map(board => [
board.name,
…cards
.filter(card => card.idBoard === board.id)
.map(card => [card.name, card.due, card.status])
])
];
const request = {
spreadsheetId,
range: ‘Sheet1!A1:D’,
valueInputOption: ‘RAW’,
resource: { values }
};
await sheets.spreadsheets.values.update(request);
console.log(‘Project dashboard updated successfully!’);
}
This example fetches data from Trello, including board names, card names, due dates, and statuses, and then writes that data to a Google Sheet. You can expand on this concept to include more data sources, perform calculations, and create dynamic visualizations.
The possibilities are endless when you start combining the Google Sheets API with other tools and services. Get creative and explore how you can streamline your workflows and unlock new insights from your data.
Advanced Use Cases and Troubleshooting
As you become more comfortable with the Google Sheets API, you may want to explore some more advanced use cases and techniques to optimize your applications.
Batch Operations
One way to improve performance is to use batch operations. Instead of making individual requests to the API, you can group multiple operations into a single request. This can significantly reduce the number of API calls and improve the overall speed of your application. Here’s an example in Python:
from googleapiclient.discovery import build
service = build(‘sheets’, ‘v4’)
# Prepare multiple requests
requests = [
{
‘updateCells’: {
‘range’: {
‘sheetId’: 0,
‘startRowIndex’: 0,
‘endRowIndex’: 10,
‘startColumnIndex’: 0,
‘endColumnIndex’: 3
},
‘fields’: ‘userEnteredValue’
}
},
{
‘addSheet’: {
‘properties’: {
‘title’: ‘New Sheet’
}
}
}
]
# Execute the batch request
response = service.spreadsheets().batchUpdate(
spreadsheetId=’YOUR_SPREADSHEET_ID’,
body={‘requests’: requests}
).execute()
print(response)
Handling Errors and Rate Limits
When working with the Google Sheets API, it’s important to handle errors and rate limits appropriately. The API can return a variety of error codes and messages, and you’ll need to be prepared to gracefully handle these situations in your application.
Here’s an example of how you might handle a rate limit error in JavaScript:
const { google } = require(‘googleapis’);
const sheets = google.sheets({ version: ‘v4’ });
async function fetchData(spreadsheetId, range) {
try {
const response = await sheets.spreadsheets.values.get({
spreadsheetId,
range
});
return response.data.values;
} catch (err) {
if (err.code === 429) {
console.log(‘Rate limit exceeded. Waiting 60 seconds before retrying…’);
await new Promise(resolve => setTimeout(resolve, 60000));
return fetchData(spreadsheetId, range);
} else {
console.error(‘Error fetching data:’, err);
throw err;
}
}
}
n this example, we check if the error code is 429 (Too Many Requests), which indicates that we’ve hit the API’s rate limit. If so, we wait 60 seconds before retrying the request. For other errors, we simply log the error and rethrow it.
Optimizing Performance
To optimize the performance of your Google Sheets API applications, you can consider techniques like:
- Caching: Store frequently accessed data in memory or a local database to reduce the number of API calls.
- Pagination: Use the pageToken parameter to fetch data in smaller chunks, rather than trying to retrieve all the data at once.
- Asynchronous Processing: Use asynchronous programming techniques to execute multiple operations concurrently, rather than waiting for each one to complete.
- Batching: As mentioned earlier, use batch operations to group multiple requests into a single API call.
By implementing these strategies, you can ensure that your applications are efficient, scalable, and responsive, even when dealing with large amounts of data.
Unlocking the Full Potential of Google Sheets
The Google Sheets API is a powerful tool that allows you to automate and integrate your spreadsheet data with a wide range of other applications and services. By mastering the techniques covered in this guide, you can unlock the full potential of your data and build innovative, data-driven applications.
Whether you’re looking to streamline your workflows, generate insights, or connect your Google Sheets data to other platforms, Coefficient’s suite of tools can help you work smarter, not harder. Get started today – it’s free.