Automated MySQL backups are crucial for maintaining data integrity and ensuring business continuity. As databases grow and become more complex, manual backups become time-consuming and prone to errors.
This guide will teach you how to automate MySQL backups effectively, covering various methods and platforms suitable for both beginners and advanced users. You’ll learn basic techniques, best practices, and modern solutions for enhanced backup management and monitoring.
MySQL Backup Automation Fundamentals
MySQL backup automation is the process of creating regular, scheduled backups of your MySQL databases without manual intervention. This practice is essential for several reasons:
- Data protection: Regular backups safeguard against data loss due to hardware failures, software errors, or human mistakes.
- Business continuity: In case of system failures, automated backups ensure quick recovery and minimal downtime.
- Compliance: Many industries require regular backups to meet regulatory standards.
Key Tools for MySQL Backup Automation
- mysqldump: A command-line utility for creating logical backups of MySQL databases.
- mysqlpump: An enhanced version of mysqldump with parallel processing capabilities.
- MySQL Enterprise Backup: A commercial tool offering advanced features like incremental and compressed backups.
Types of MySQL Backups
- Full backups: Complete copies of the entire database, ideal for smaller datasets.
- Incremental backups: Only back up data that has changed since the last backup, saving time and storage space.
- Differential backups: Back up all changes since the last full backup, offering a balance between full and incremental backups.
Choosing the Right Backup Strategy
Selecting the appropriate backup strategy depends on several factors:
- Database size: Larger databases may benefit from incremental or differential backups to reduce backup time and storage requirements.
- Recovery time objectives (RTO): Consider how quickly you need to restore data in case of failure.
- Available resources: Assess your storage capacity and processing power to determine the most suitable backup method.
- Regulatory requirements: Ensure your backup strategy complies with industry-specific regulations.
Automate MySQL Backups on Linux: Cron Jobs and Bash Scripts
Linux systems offer powerful tools for automating MySQL backups using cron jobs and bash scripts. Here’s a step-by-step guide to implement a simple automated backup solution:
Create a backup script:
#!/bin/bash
# Set variables
BACKUP_DIR=”/path/to/backup/directory”
MYSQL_USER=”your_mysql_user”
MYSQL_PASSWORD=”your_mysql_password”
DATABASE_NAME=”your_database_name”
DATE=$(date +”%Y-%m-%d_%H-%M-%S”)
# Create backup
mysqldump -u $MYSQL_USER -p$MYSQL_PASSWORD $DATABASE_NAME > $BACKUP_DIR/$DATABASE_NAME-$DATE.sql
# Compress backup
gzip $BACKUP_DIR/$DATABASE_NAME-$DATE.sql
# Delete backups older than 7 days
- find $BACKUP_DIR -name “*.sql.gz” -mtime +7 -delete
- Make the script executable:
chmod +x /path/to/backup_script.sh - Set up a cron job:
crontab -e
Add the following line to run the backup daily at 2 AM:
0 2 * * * /path/to/backup_script.sh - Best practices for file naming and storage:
- Use descriptive names including the database name and timestamp.
- Store backups in a separate directory or drive.
- Implement rotation to manage storage space.
By automating MySQL backups on Linux, you ensure regular, consistent backups without manual intervention.
Windows MySQL Backup Automation: Task Scheduler and PowerShell
Windows users can leverage Task Scheduler and PowerShell to automate MySQL backups. Here’s how to set it up:
Create a PowerShell script for MySQL backup:
# Set variables
$backupDir = “C:\MySQLBackups”
$mysqlUser = “your_mysql_user”
$mysqlPassword = “your_mysql_password”
$databaseName = “your_database_name”
$date = Get-Date -Format “yyyy-MM-dd_HH-mm-ss”
# Create backup
& “C:\Program Files\MySQL\MySQL Server 8.0\bin\mysqldump.exe” -u $mysqlUser -p$mysqlPassword $databaseName > “$backupDir\$databaseName-$date.sql”
# Compress backup
Compress-Archive -Path “$backupDir\$databaseName-$date.sql” -DestinationPath “$backupDir\$databaseName-$date.zip”
# Delete original SQL file
Remove-Item “$backupDir\$databaseName-$date.sql”
# Delete backups older than 7 days
- Get-ChildItem -Path $backupDir -Filter “*.zip” | Where-Object { $_.LastWriteTime -lt (Get-Date).AddDays(-7) } | Remove-Item
- Set up Windows Task Scheduler:
- Open Task Scheduler and create a new task.
- Set the trigger to run daily at your preferred time.
- Add an action to start a program: powershell.exe
- Set the argument: -ExecutionPolicy Bypass -File “C:\Path\To\Your\BackupScript.ps1”
Integrate with Windows Event Viewer for logging: Add the following lines to your PowerShell script to log events:
# Log backup event
- Write-EventLog -LogName Application -Source “MySQL Backup” -EventId 1000 -EntryType Information -Message “MySQL backup completed successfully for $databaseName”
- Troubleshooting common issues:
- Ensure PowerShell execution policy allows running scripts.
- Check file permissions for the backup directory.
- Verify MySQL credentials and connection details.
Docker-based MySQL Backup Automation: Containerized Solutions
Automating MySQL backups in Docker environments requires a slightly different approach. Here’s a guide to setting up automated backups for containerized MySQL databases:
Create a backup script:
#!/bin/bash
# Set variables
MYSQL_CONTAINER=”your_mysql_container_name”
BACKUP_DIR=”/path/to/backup/directory”
DATE=$(date +”%Y-%m-%d_%H-%M-%S”)
# Create backup
docker exec $MYSQL_CONTAINER mysqldump -u root -p”$MYSQL_ROOT_PASSWORD” –all-databases > $BACKUP_DIR/all-databases-$DATE.sql
# Compress backup
gzip $BACKUP_DIR/all-databases-$DATE.sql
# Delete backups older than 7 days
find $BACKUP_DIR -name “*.sql.gz” -mtime +7 -delete
Create a Docker volume for backup storage:
docker volume create mysql-backups
Implement backup rotation in containers: Add the following to your Docker Compose file:
services:
mysql:
image: mysql:latest
volumes:
– mysql-data:/var/lib/mysql
– mysql-backups:/backups
backup:
image: alpine
volumes:
– mysql-backups:/backups
command: |
/bin/sh -c “
apk add –no-cache mysql-client
while true; do
mysqldump -h mysql -u root -p$$MYSQL_ROOT_PASSWORD –all-databases > /backups/all-databases-$$(date +%Y-%m-%d_%H-%M-%S).sql
find /backups -name ‘*.sql’ -mtime +7 -delete
sleep 86400
done
“
volumes:
mysql-data:
mysql-backups:
Best practices for securing containerized MySQL backups:
- Use Docker secrets to manage sensitive information like passwords.
- Implement network isolation for your backup containers.
- Regularly update your Docker images to patch security vulnerabilities.
By following these steps, you can ensure that your containerized MySQL databases are regularly backed up and secured.
Advanced MySQL Backup Automation Techniques and Best Practices
As your MySQL infrastructure grows, you may need to implement more sophisticated backup strategies. Here are some advanced techniques and best practices:
Automate Backups for Distributed MySQL Systems and Multi-node Clusters
- Use a centralized backup server:
- Set up a dedicated server to handle backups for all nodes.
- Implement a script that connects to each node and initiates backups.
- Leverage replication for backups:
- Set up a backup slave that’s always slightly behind the master.
- Take backups from the slave to reduce load on the production system.
Implement Point-in-Time Recovery with Binary Log Backups
Enable binary logging in your MySQL configuration:
[mysqld]
log-bin = mysql-bin
binlog_expire_logs_seconds = 604800 # 7 days
Create a script to backup binary logs:
#!/bin/bash
MYSQL_DATA_DIR=”/var/lib/mysql”
BACKUP_DIR=”/path/to/binlog/backups”
# Flush and copy current binary log
mysql -e “FLUSH BINARY LOGS”
# Copy all binary logs except the current one
- find $MYSQL_DATA_DIR -name “mysql-bin.*” ! -name “mysql-bin.index” ! -name “mysql-bin.$(cat $MYSQL_DATA_DIR/mysql-bin.index | tail -n 1)” -exec cp {} $BACKUP_DIR \;
- Schedule this script to run regularly alongside your full backups.
Optimize Backup Performance and Resource Usage
- Use compression to reduce backup size and transfer time:
mysqldump -u root -p –all-databases | gzip > backup.sql.gz - Implement parallel backups for large databases:
mysqldump -u root -p –all-databases –skip-lock-tables | pigz > backup.sql.gz - Schedule backups during off-peak hours to minimize impact on production systems.
Implement Version Control for Backup Scripts and Configurations
Use Git to version control your backup scripts and configurations:
git init
git add backup_script.sh mysql_config.cnf
- git commit -m “Initial backup configuration”
- Store your Git repository on a separate server or use a service like GitHub (private repository) for added redundancy.
Test and Validate Automated MySQL Backups
Regular testing and validation of your automated MySQL backups are crucial to ensure that your data can be recovered when needed. Here’s a comprehensive approach to testing and validating your backups:
Importance of Regular Backup Testing
- Verify backup integrity: Ensure that backups are complete and uncorrupted.
- Validate recovery processes: Confirm that your restoration procedures work as expected.
- Meet compliance requirements: Many regulations require regular testing of backup and recovery processes.
- Identify potential issues: Discover and address problems before they impact your ability to recover data.
Methods for Verifying Backup Integrity
Checksum verification:
mysqldump –all-databases | md5sum > backup_checksum.md5
Compare this checksum with one generated from the backup file to ensure integrity.
Sample data verification: Restore a small subset of data and compare it with the live database:
SELECT COUNT(*) FROM sample_table;
Run this on both the live and restored databases to compare record counts.
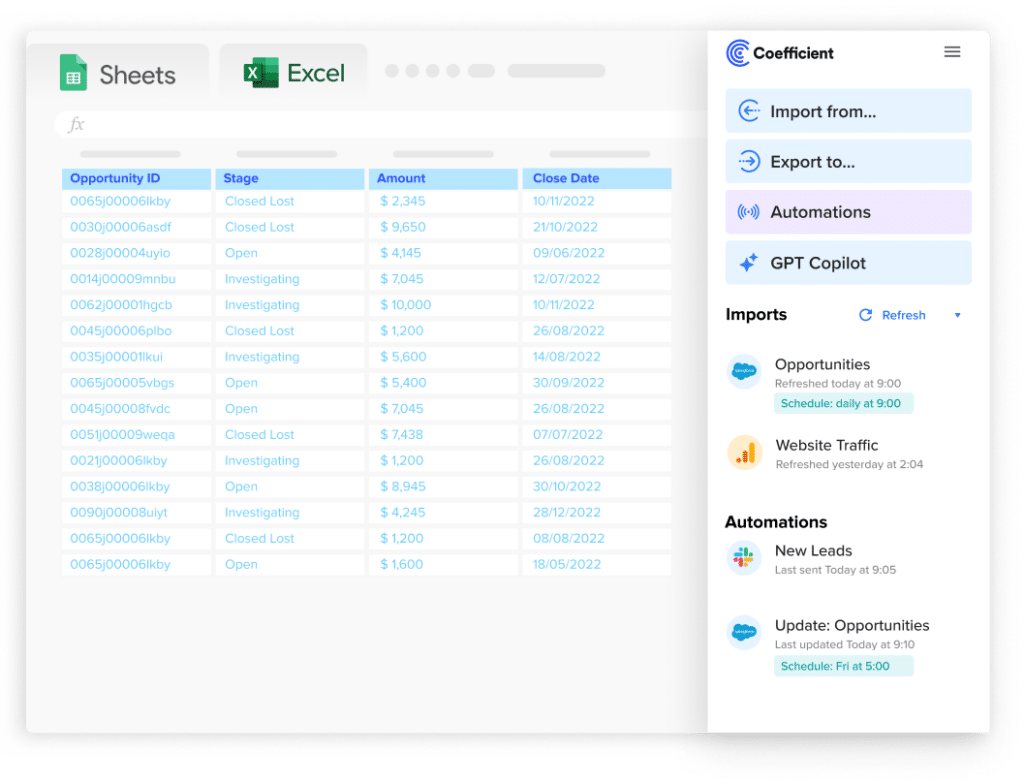
Stop exporting data manually. Sync data from your business systems into Google Sheets or Excel with Coefficient and set it on a refresh schedule.
Get Started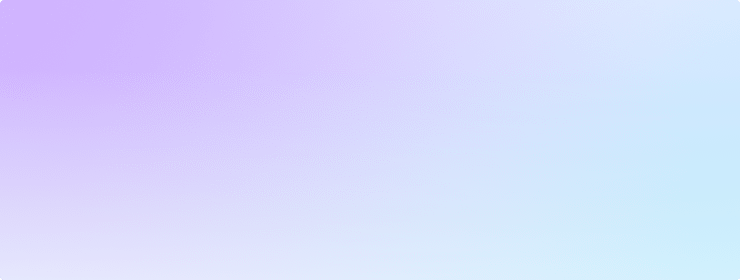
Automated integrity checks: Create a script that performs basic checks on your backup files:
#!/bin/bash
BACKUP_FILE=$1
# Check if file exists and is not empty
if [ ! -s “$BACKUP_FILE” ]; then
echo “Backup file is empty or does not exist”
exit 1
fi
# Check if file is readable
if [ ! -r “$BACKUP_FILE” ]; then
echo “Backup file is not readable”
exit 1
fi
# For compressed backups, check if they can be decompressed
if [[ “$BACKUP_FILE” == *.gz ]]; then
if ! gzip -t “$BACKUP_FILE”; then
echo “Backup file is corrupted”
exit 1
fi
fi
echo “Backup file passed basic integrity checks”
Simulate Recovery Scenarios
- Full database restoration: Regularly perform a complete restoration of your database to a test environment:
gunzip < backup.sql.gz | mysql -u root -p test_database - Point-in-time recovery: Test your ability to restore the database to a specific point in time using binary logs:
mysqlbinlog mysql-bin.000001 mysql-bin.000002 | mysql -u root -p - Partial data recovery: Practice restoring specific tables or data ranges:
mysqldump -u root -p –databases db_name –tables table_name | mysql -u root -p test_database
Automate Backup Validation Processes
Create a validation script:
#!/bin/bash
BACKUP_FILE=$1
TEST_DB=”test_restore_db”
# Restore backup to test database
mysql -u root -p -e “CREATE DATABASE IF NOT EXISTS $TEST_DB”
gunzip < $BACKUP_FILE | mysql -u root -p $TEST_DB
# Run validation queries
mysql -u root -p $TEST_DB -e “SELECT COUNT(*) FROM important_table” > count_result.txt
# Compare results with expected values
if grep -q “expected_count” count_result.txt; then
echo “Backup validation successful”
else
echo “Backup validation failed”
fi
# Clean up
mysql -u root -p -e “DROP DATABASE $TEST_DB”
- rm count_result.txt
- Schedule regular validation: Add this script to your cron jobs or task scheduler to run after each backup.
Compliance and Security in MySQL Backup Automation
Ensuring compliance and security in your MySQL backup automation process is crucial for protecting sensitive data and meeting regulatory requirements. Here’s a comprehensive approach to addressing these concerns:
Address Regulatory Requirements (GDPR, HIPAA, etc.)
- Data retention policies:
- Implement backup retention schedules that comply with regulations.
- Create a script to automatically delete backups older than the required retention period
find /backup/directory -name “*.sql.gz” -mtime +365 -delete
- Data access controls:
- Limit access to backup files and restoration processes.
- Use role-based access control (RBAC) for backup management.
- Audit trails:
- Log all backup and restore activities.
Create a logging function in your backup scripts:
log_activity() {
echo “$(date): $1” >> /var/log/mysql_backup.log
}
Implement Encryption for Backup Files
- Use MySQL’s built-in encryption
mysqldump -u root -p –all-databases –encrypt-password=your_encryption_key > encrypted_backup.sql - Encrypt backup files after creation:
openssl enc -aes-256-cbc -in backup.sql -out backup.sql.enc -pass pass:your_encryption_key - Manage encryption keys securely:
- Use a key management system (KMS) to store and rotate encryption keys.
- Avoid storing encryption keys in plain text or version control systems.
Manage Access Control for Automated Backup Systems
- Use least privilege principle:
Create a dedicated MySQL user for backups with minimal required permissions:
CREATE USER ‘backup_user’@’localhost’ IDENTIFIED BY ‘strong_password’;
GRANT SELECT, LOCK TABLES, SHOW VIEW, EVENT, TRIGGER ON *.* TO ‘backup_user’@’localhost’;
- FLUSH PRIVILEGES;
- Implement network-level security:
- Use firewalls to restrict access to backup servers.
- Employ VPNs client for remote backup management.
- Secure backup storage:
- Use encrypted file systems for backup storage.
- Implement multi-factor authentication for accessing backup storage systems.
Troubleshooting Common MySQL Backup Automation Issues
Even with a well-designed backup system, issues can arise. Here’s how to identify and resolve common problems in MySQL backup automation:
Identify and Resolve Backup Failures
Check MySQL error logs:
tail -f /var/log/mysql/error.log
Verify MySQL user permissions:
SHOW GRANTS FOR ‘backup_user’@’localhost’;
Ensure sufficient disk space:
df -h
Test MySQL connectivity:
mysqladmin ping -u backup_user -p
Handle Incomplete or Corrupted Backups
Implement checksum verification:
mysqldump –all-databases | tee >(md5sum > backup.md5) > backup.sql
Use mysqlcheck for database integrity:
mysqlcheck –all-databases –check –repair
Implement redundant backups to different locations.
Address Performance Issues During Backup Processes
Monitor system resources during backups:
top -b -n 1 > resource_usage.log
Use less resource-intensive backup methods:
- Consider incremental backups for large databases
- Use compression to reduce I/O:
mysqldump –all-databases | gzip > backup.sql.gz
Schedule backups during off-peak hours.
Secure Your Data with Automated MySQL Backups
Automating MySQL backups is crucial for data protection and business continuity. But managing backups across platforms and monitoring their status can be complex.
Coefficient connects your MySQL data directly to spreadsheets, simplifying backup monitoring and analysis.
Get started with Coefficient today and discover how our solutions can transform your approach to data management and analysis.