SQL optimization is crucial for improving database performance and query efficiency. By implementing these advanced techniques, you can significantly enhance your database operations and reduce query execution time. Let’s explore the top five SQL optimization hacks that professionals use to boost their database performance.
1. Strategic Query Restructuring
Effective query restructuring can dramatically improve SQL performance. Here are three key strategies to optimize your queries:
Replace SELECT * with Specific Column Names
Instead of using SELECT *, which retrieves all columns from a table, specify only the columns you need. This practice reduces the amount of data transferred and processed, leading to faster query execution.
Steps to implement:
- Identify the specific columns required for your query.
- Replace SELECT * with SELECT column1, column2, column3.
- Ensure all necessary columns are included to avoid multiple queries.
Example:
— Instead of:
SELECT * FROM customers
— Use:
SELECT customer_id, first_name, last_name, email FROM customers
Optimize Subqueries and Complex JOINs
Complex queries with multiple subqueries or JOINs can slow down performance. Optimize these by simplifying the query structure and using appropriate JOIN types.
Steps to optimize:
- Analyze the query execution plan to identify bottlenecks.
- Consider replacing subqueries with JOINs where possible.
- Use the appropriate JOIN type (INNER, LEFT, RIGHT) based on your data requirements.
- Ensure proper indexing on JOIN columns.
Example:
— Instead of:
SELECT o.order_id, o.order_date
FROM orders o
WHERE o.customer_id IN (SELECT customer_id FROM customers WHERE country = ‘USA’)
— Use:
SELECT o.order_id, o.order_date
FROM orders o
INNER JOIN customers c ON o.customer_id = c.customer_id
WHERE c.country = ‘USA’
Use WHERE Instead of HAVING for Filtering
The WHERE clause filters rows before grouping, while HAVING filters after grouping. Using WHERE when possible can improve query performance by reducing the amount of data processed.
Steps to implement:
- Identify filtering conditions in your query.
- Move conditions that don’t involve aggregates to the WHERE clause.
- Keep conditions involving aggregates in the HAVING clause.
Example:
— Instead of:
SELECT department, AVG(salary)
FROM employees
GROUP BY department
HAVING department = ‘Sales’
— Use:
SELECT department, AVG(salary)
FROM employees
WHERE department = ‘Sales’
GROUP BY department
2. Advanced Indexing Techniques
Proper indexing is essential for SQL optimization. Here are three advanced indexing techniques to enhance query performance:
Implement Covering Indexes for Query Optimization
A covering index includes all the columns required by a query, allowing the database to retrieve data directly from the index without accessing the table.
Steps to create a covering index:
- Identify frequently used queries that could benefit from a covering index.
- Analyze the columns used in the SELECT, WHERE, and ORDER BY clauses.
- Create an index that includes all these columns.
Example:
CREATE INDEX idx_covering ON orders (order_date, customer_id, total_amount);
— This query can now use the covering index:
SELECT order_date, total_amount
FROM orders
WHERE customer_id = 123
ORDER BY order_date;
Balance Index Creation and Maintenance
While indexes improve query performance, too many indexes can slow down write operations and increase storage requirements.
Steps to balance index creation:
- Regularly analyze query performance and index usage.
- Remove or consolidate redundant indexes.
- Consider the trade-off between read and write performance when creating new indexes.
- Use database monitoring tools to track index usage and performance impact.
Identify and Remove Unused Indexes
Unused indexes consume storage space and can negatively impact write performance without providing any benefits.
Steps to remove unused indexes:
- Use database-specific tools or queries to identify unused indexes.
- Analyze the impact of removing each unused index.
- Create a backup of the index definition before removal.
- Remove the unused index and monitor query performance.
Example (for MySQL):
SELECT * FROM sys.schema_unused_indexes;
3. Query Execution Plan Analysis
Analyzing query execution plans is crucial for identifying performance bottlenecks and optimizing SQL queries.
Leverage EXPLAIN Command for Performance Tuning
The EXPLAIN command provides valuable information about how the database executes a query, including the order of table access and the use of indexes.
Steps to use EXPLAIN:
- Prefix your SQL query with the EXPLAIN keyword.
- Run the command and analyze the output.
- Look for full table scans, inefficient join orders, or missing index usage.
- Adjust your query or database structure based on the findings.
Example:
sql
Copy
EXPLAIN SELECT * FROM orders WHERE customer_id = 123;
Identify and Resolve Query Plan Bottlenecks
Once you’ve obtained the execution plan, focus on resolving any bottlenecks identified.
Steps to resolve bottlenecks:
- Look for operations with high cost or large row counts.
- Identify missing indexes or inefficient join conditions.
- Consider rewriting the query to avoid expensive operations.
- Test alternative query structures and compare execution plans.
Utilize Plan Guides for Complex Queries
Plan guides allow you to influence the query optimizer’s decisions for complex queries that are difficult to optimize through standard methods.
Steps to use plan guides:
- Identify a query that consistently performs poorly.
- Create a plan guide specifying the desired execution plan.
- Test the query with the plan guide applied.
- Monitor performance and adjust the plan guide as needed.
Example (for SQL Server):
EXEC sp_create_plan_guide
@name = N’Guide1′,
@stmt = N’SELECT * FROM orders WHERE customer_id = @CustomerID’,
@type = N’SQL’,
@module_or_batch = NULL,
@params = N’@CustomerID int’,
@hints = N’OPTION (FORCESCAN)’;
4. Data Access Optimization
Optimizing data access patterns can significantly improve SQL performance, especially for large datasets.
Minimize Table Scans with Effective Indexing
Table scans can be resource-intensive, especially for large tables. Effective indexing can help avoid unnecessary scans.
Steps to minimize table scans:
- Identify queries that frequently perform full table scans.
- Analyze the WHERE, JOIN, and ORDER BY clauses of these queries.
- Create appropriate indexes to support these clauses.
- Use covering indexes where possible to avoid accessing the table data.
Example:
CREATE INDEX idx_customer_order ON orders (customer_id, order_date);
— This query can now use the index instead of a table scan:
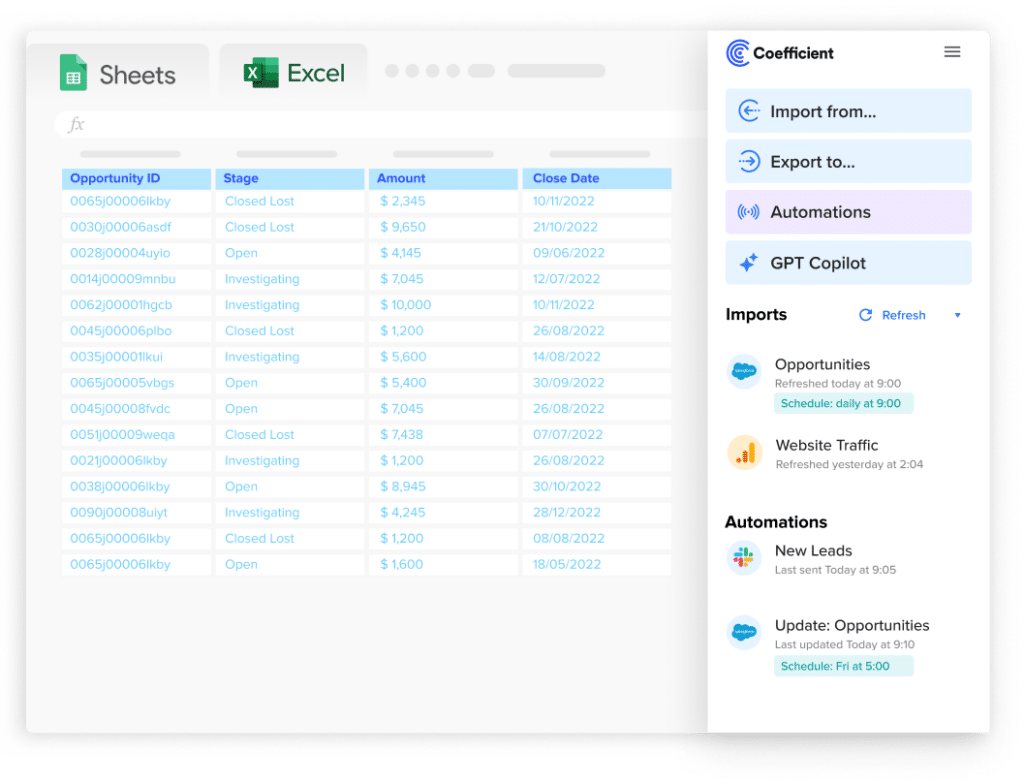
Stop exporting data manually. Sync data from your business systems into Google Sheets or Excel with Coefficient and set it on a refresh schedule.
Get Started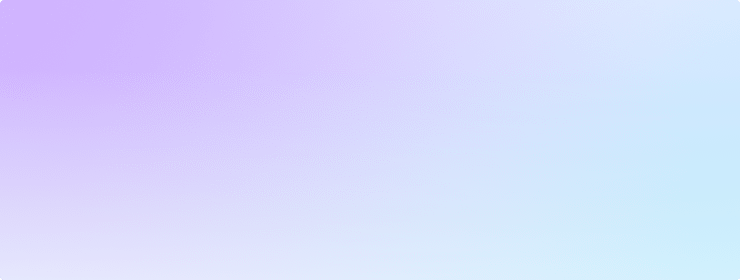
SELECT * FROM orders WHERE customer_id = 123 ORDER BY order_date;
Implement Partitioning for Large Datasets
Partitioning divides large tables into smaller, more manageable pieces, improving query performance and data management.
Steps to implement partitioning:
- Identify tables that would benefit from partitioning (e.g., large tables with clear partition keys).
- Choose an appropriate partitioning strategy (range, list, hash).
- Create the partitioned table and transfer data.
- Adjust queries to take advantage of partition pruning.
Example (for PostgreSQL):
CREATE TABLE orders (
order_id INT,
order_date DATE,
customer_id INT,
total_amount DECIMAL(10,2)
) PARTITION BY RANGE (order_date);
CREATE TABLE orders_2023 PARTITION OF orders
FOR VALUES FROM (‘2023-01-01’) TO (‘2024-01-01’);
CREATE TABLE orders_2024 PARTITION OF orders
FOR VALUES FROM (‘2024-01-01’) TO (‘2025-01-01’);
Leverage In-Memory OLTP Techniques for Critical Operations
In-memory OLTP can significantly improve performance for critical operations by storing and processing data entirely in memory.
Steps to implement in-memory OLTP:
- Identify tables and stored procedures that would benefit from in-memory optimization.
- Create memory-optimized tables and natively compiled stored procedures.
- Migrate data to memory-optimized tables.
- Adjust application code to use memory-optimized objects.
Example (for SQL Server):
CREATE TABLE dbo.InMemoryOrders
(
OrderID INT IDENTITY PRIMARY KEY NONCLUSTERED,
CustomerID INT NOT NULL INDEX ix_CustomerID,
OrderDate DATETIME2 NOT NULL,
TotalAmount MONEY
)
WITH (MEMORY_OPTIMIZED = ON);
5. Query Writing Best Practices
Adhering to query writing best practices can lead to significant performance improvements.
Optimize Wildcard Usage in LIKE Operations
Wildcard searches, especially those with leading wildcards, can be slow. Optimize these operations to improve performance.
Steps to optimize wildcard usage:
- Avoid using leading wildcards when possible.
- Consider using full-text search for complex pattern matching.
- Use appropriate indexes to support LIKE operations.
Example:
— Instead of:
SELECT * FROM customers WHERE last_name LIKE ‘%son’
— Use:
SELECT * FROM customers WHERE last_name LIKE ‘son%’
Effective Use of LIMIT for Result Sampling
The LIMIT clause can improve performance by reducing the amount of data returned.
Steps to use LIMIT effectively:
- Identify queries that return large result sets.
- Add a LIMIT clause to restrict the number of rows returned.
- Ensure proper ordering when using LIMIT.
Example:
SELECT * FROM orders
ORDER BY order_date DESC
LIMIT 100;
Avoid Unnecessary DISTINCT Operations
The DISTINCT keyword can be computationally expensive. Avoid using it unnecessarily.
Steps to optimize DISTINCT usage:
- Analyze queries using DISTINCT to determine if it’s truly necessary.
- Consider using GROUP BY as an alternative.
- Ensure proper indexing to support DISTINCT operations when needed.
Example:
— Instead of:
SELECT DISTINCT customer_id FROM orders
— Use:
SELECT customer_id FROM orders GROUP BY customer_id
Optimize SQL Queries for Faster Results
Advanced SQL techniques can significantly boost database performance. But managing complex data structures across multiple sources can be challenging.
Coefficient simplifies SQL optimization with features like JSON Flattening,which allows you to neatly expand and flatten nested JSON fields during data import. This feature enhances the richness of data points across various connectors, making it easier to work with complex data structures in your SQL queries.
Get started with Coefficient and transform your approach to SQL optimization and data analysis.